1、创建项目
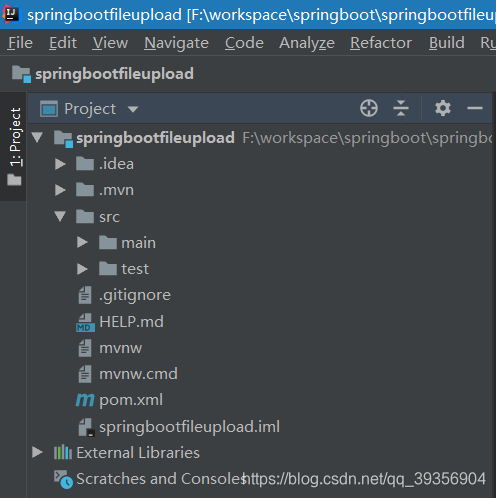
2、POM文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.6.RELEASE</version> <relativePath/> </parent> <groupId>com.xiezhenyu</groupId> <artifactId>springbootfileupload</artifactId> <version>0.0.1-SNAPSHOT</version> <name>springbootfileupload</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
|
3、启动类
1 2 3 4 5 6 7 8 9
| package com.xiezhenyu.springbootfileupload; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class SpringbootfileuploadApplication { public static void main(String[] args) { SpringApplication.run(SpringbootfileuploadApplication.class, args); } }
|
4、编写上传页面
1 2 3 4 5 6 7 8 9 10
| <html> <head> </head> <body> <form action="/fileUploadController" method="post" enctype="multipart/form-data"> <input type="file" name="file"/> <input type="submit" value="okok"/> </form> </body> </html>
|
5、编写Controller
注意:在文件上传方法中,参数的名字必须和html中的name相同。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.xiezhenyu.springbootfileupload.controller; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.multipart.MultipartFile; import java.io.File; @RestController public class FileUploadController {
@PostMapping("/fileUploadController") public String fileUpload(MultipartFile file) throws Exception{ System.out.println(file.getOriginalFilename()); file.transferTo(new File("d:/"+file.getOriginalFilename())); return "yes"; } }
|
6、修改上传文件大小
默认上传的大小为1M一下,要使上传大小变化必须修改application.properties配置文件。
1 2 3 4
| #配置单个上传文件的大小限制 spring.servlet.multipart.max-file-size=2MB #配置一次请求(批量)上传文件的总大小 spring.servlet.multipart.max-request-size=20MB
|