Josephu(约瑟夫、约瑟夫环)问题:
设编号为1,2,…,n的n个人围坐一圈,约定的编号为k(1<=k<=n)的人从1开始报数,数到的那个人又出列,依此类推,知道所有人出列为止,由此产生一个出队编号的序列。
用一个不带头节点的循环链表来处理Josepho问题:
先构成一个有n个节点的单循环链表,然后由k节点起从1开始计数,计到m时,对应节点从链表中删除,直到最后一个节点从链表中删除算法结束。
单向环形链表的介绍
链表形成一个环状
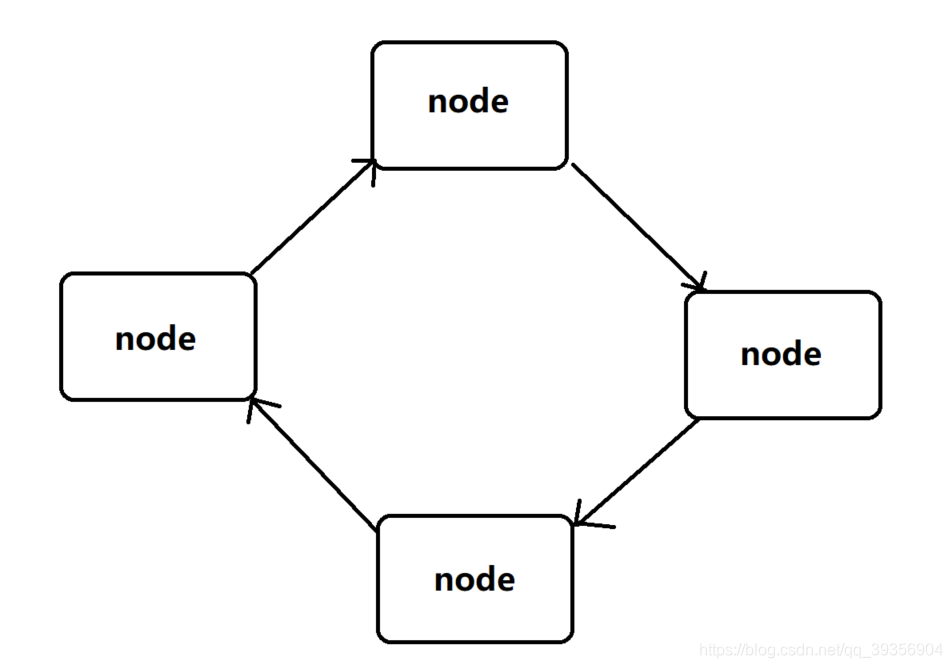
约瑟夫问题的图释
Josephu(约瑟夫、约瑟夫环)问题:
设编号为1,2,…,n的n个人围坐一圈,约定的编号为k(1<=k<=n)的人从1开始报数,数到的那个人又出列,依此类推,知道所有人出列为止,由此产生一个出队编号的序列。
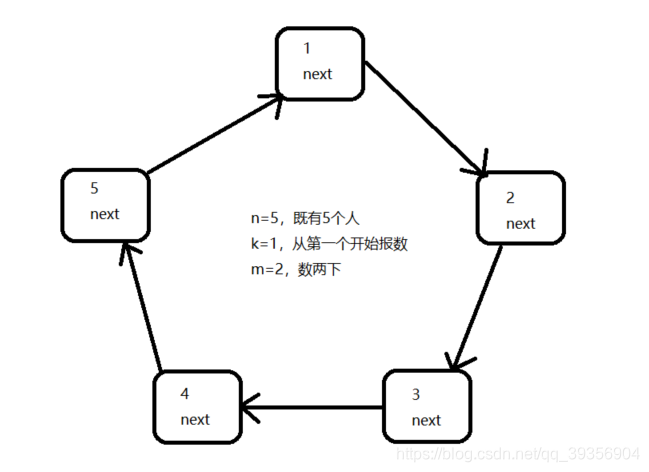
以上图的出队顺序为:
2->4->1->5->3
约瑟夫问题的代码实现
思路:
构建一个环形链表
[1]创建第一个节点,让一个指针(变量)first指向该节点,并形成一个环形
[2]后面当我们每创建一个节点,就把该节点,加入到已有的环形链表中即可
遍历环形链表
[1]先让一个辅助指针(变量)curBoy,指向first节点
[2]然后通过一个while循环遍历该环形链表即可
[3]当curBoy.next == first 遍历结束
代码:
书写节点类Boy
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| class Boy { private int no; private Boy next; public Boy(int no){ this.no=no; } public int getNo() { return no; } public void setNo(int no) { this.no = no; } public Boy getNext() { return next; } public void setNext(Boy next) { this.next = next; } }
|
创建一个环形单向链表
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| class CircleSingkeLinkedList { private Boy first = null; public void addBoy(int nums){ if(nums<1){ System.out.println("nums的值不正确"); return; } Boy curBoy = null; for(int i = 1;i <= nums; i++) { Boy boy = new Boy(i); if(i==1) { first = boy; first.setNext(first); curBoy = first; } else { curBoy.setNext(boy); boy.setNext(first); curBoy = boy; } } } public void showBoy() { if(first==null) { System.out.println("链表为空!");return; } Boy curBoy = first; while(true) { System.out.printf("小孩的编号%d\n",curBoy.getNo()); if(curBoy.getNext()==first)break; curBoy = curBoy.getNext(); } } }
|
根据用户的输入,生成一个小孩出圈的顺序
[1]需要创建一个辅助指针(变量)helper,事先应该指向环形链表的最后这个节点。
[2]小孩报数前,先让first和helper移动k-1次
[2]当小孩报数时,让first和helper同时移动m-1次
[3]这时就可以将first指向的小孩节点出圈
first=first.next
helper.next=first
[4]原来first指向的这个节点就没有任何引用了
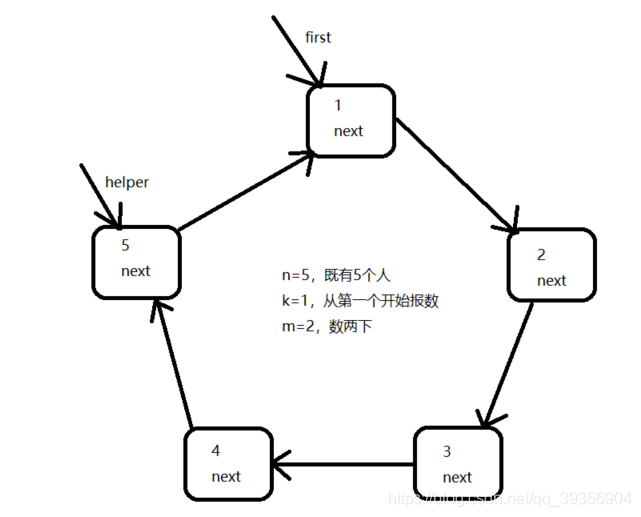
向环形单向链表类CircleSingkeLinkedList中添加出圈这个方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
|
public void countBoy(int startNo,int countNum,int nums) { if(first==null||startNo<1||startNo>nums){ System.out.println("参数输入有误!");return; } Boy helper = first; while(true) { if(helper.getNext() == first) { break; } helper = helper.getNext(); } for(int j = 0;j<startNo - 1;j++){ first = first.getNext(); helper = helper.getNext(); } while(true) { if(helper == first)break; for(int j = 0;j<countNum-1;j++) { first = first.getNext(); helper = helper.getNext(); } System.out.printf("小孩%d出圈\n",first.getNo()); first = first.getNext(); helper.setNext(first); } System.out.printf("最后留在圈中的小孩编号%d\n",first.getNo()); }
|
测试:
1 2 3 4 5 6
| public static void main(String[] args) { CircleSingkeLinkedList c = new CircleSingkeLinkedList(); c.addBoy(5); c.countBoy(1, 2, 5); }
|
结果:
1 2 3 4 5
| 小孩2出圈 小孩4出圈 小孩1出圈 小孩5出圈 最后留在圈中的小孩编号3
|